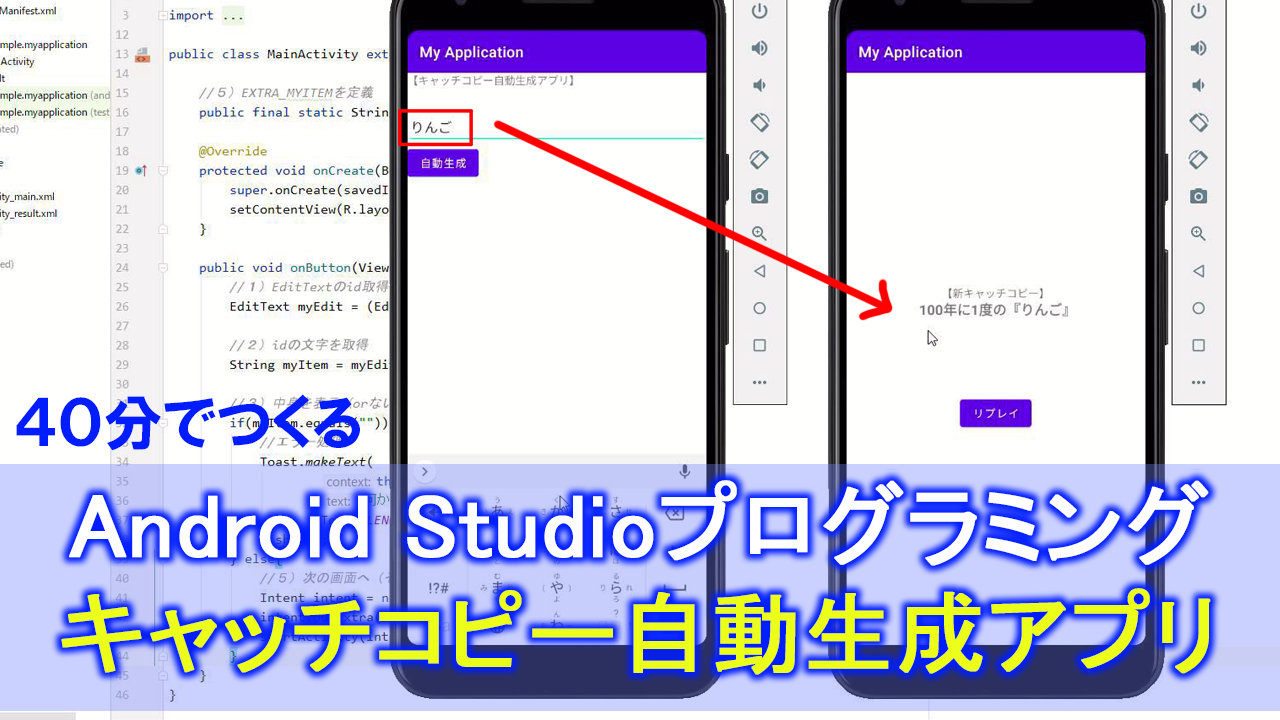
AndroidStudioで、「キャッチコピー自動生成アプリ」を作っていきます。
主な学習のテーマは
「Intent」(画面遷移)と
「putExtra」(情報の受け渡し)
です。
▼作業環境
OS:win10
言語:java
AndroidStudio:4.1.1
▼主な対象者
javaの入門的な構文は少しかじったので、実際何か「モノ」を作って成果を体験してみたい人
動画(実況解説)
テキスト
1)activity.main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="【キャッチコピー自動生成アプリ】" /> <EditText android:id="@+id/et" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="40dp" android:hint="アイテムを入力してね" /> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="onButton" android:text="自動生成" /> </LinearLayout>
2)MainActivity.java
package com.example.mycatchphrase; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.EditText; import android.widget.Toast; public class MainActivity extends AppCompatActivity { //5)EXTRA_MYITEMを定義 public final static String EXTRA_MYITEM ="com.example.mycatchphrase.EXTRA_MYITEM"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void onButton(View view){ //1)EditTextのid取得 EditText myEdit = (EditText)findViewById(R.id.et); //2)idの文字を取得 String myItem =myEdit.getText().toString(); //3)中身を表示(orない場合はエラー処理) if(myItem.equals("")){ //エラー処理 Toast.makeText( this, "何か書いて", Toast.LENGTH_LONG ).show(); }else{ //5)次の画面へ(4その前に次の画面を作る) Intent intent = new Intent(this,Result.class); intent.putExtra(EXTRA_MYITEM,myItem); startActivity(intent); } } }
3)activity_result.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical" tools:context=".Result"> <!--4)画面作成--> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="【新キャッチコピー】" /> <TextView android:id="@+id/itemResult" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="●●です" android:textSize="18sp" android:textStyle="bold" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="100dp" android:onClick="onReplay" android:text="リプレイ" /> </LinearLayout>
4)Result.java
package com.example.mycatchphrase; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.TextView; import java.util.Random; public class Result extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_result); //6)intentのデータを取得 Intent intent =getIntent(); String myItem = intent.getStringExtra(MainActivity.EXTRA_MYITEM); //7)TextViewに表示 TextView itemResult =(TextView)findViewById(R.id.itemResult); //itemResult.setText(myItem + "です"); //8)配列を用意 String [] str ={ " 女子高生が選ぶ『" + myItem + "』ランキング", "100円ショップにあるものだけで『" + myItem +"』を作る", " 世界の富豪が実践する3つの『" + myItem + "』", " 逆に今『" + myItem + "』がナウい!", " 世界は『" + myItem + "』で出来ている", " 彼のハートを打ち抜く神『" + myItem + "』", " 衝撃!『" + myItem + "』だけで1ヵ月生活", " あなたの知らない『" + myItem + "』の世界", " 今からはじめる『" + myItem + "』", " 乳酸菌が100億個入った『" + myItem + "』", " 100年に1度の『" + myItem + "』", " 愛と『" + myItem + "』の物語", " 世界よ!これが『" + myItem + "』だ!!", " あの『" + myItem + "』、待望の映画化!", " 今年最高の『" + myItem + "』をお届け", }; //10)ランダムで数字を取得 int strNum =str.length; Random random = new Random(); int ranNum =random.nextInt(strNum); //9)配列を表示 //11)ランダムに表示 itemResult.setText(str[ranNum]); } public void onReplay(View view){ finish(); } }