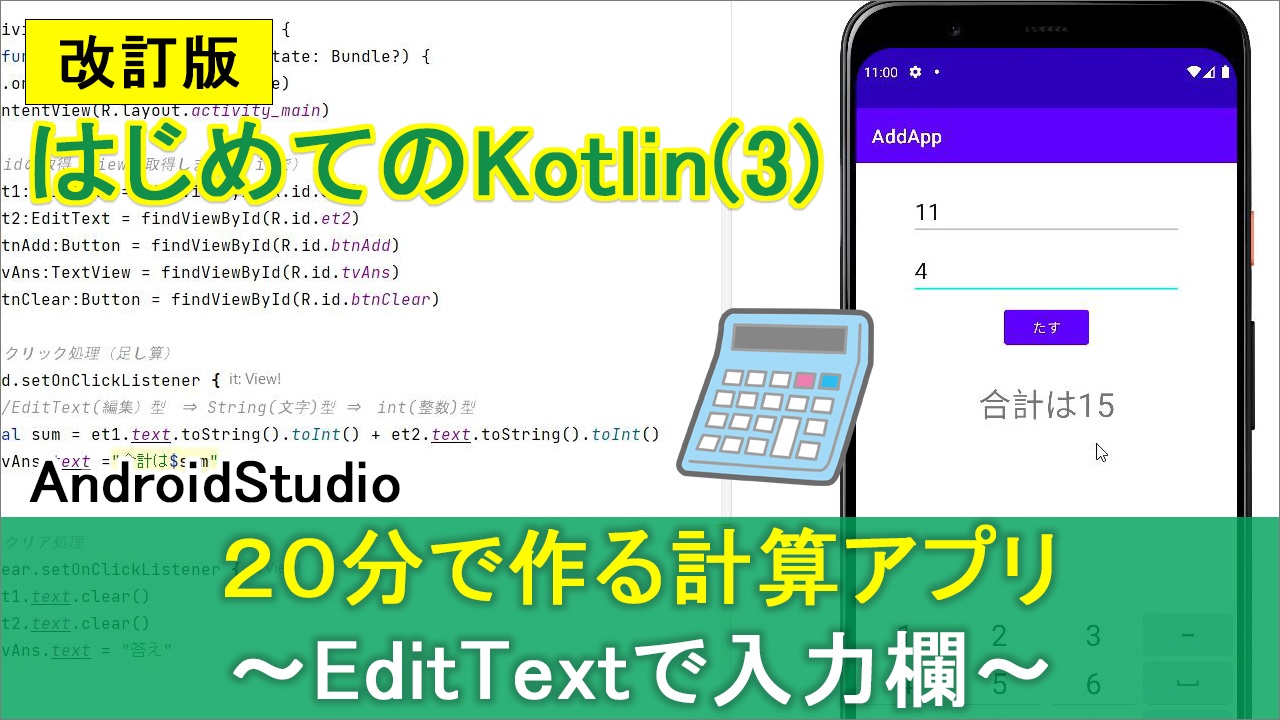
※注意)2023年6月以降のバージョンでは「Empty Views Activity」という名称に変わったようです
動画内では「EmptyActivity」と紹介していますが、新バージョンでは「Empty Views Activity」に名称が変わったようです
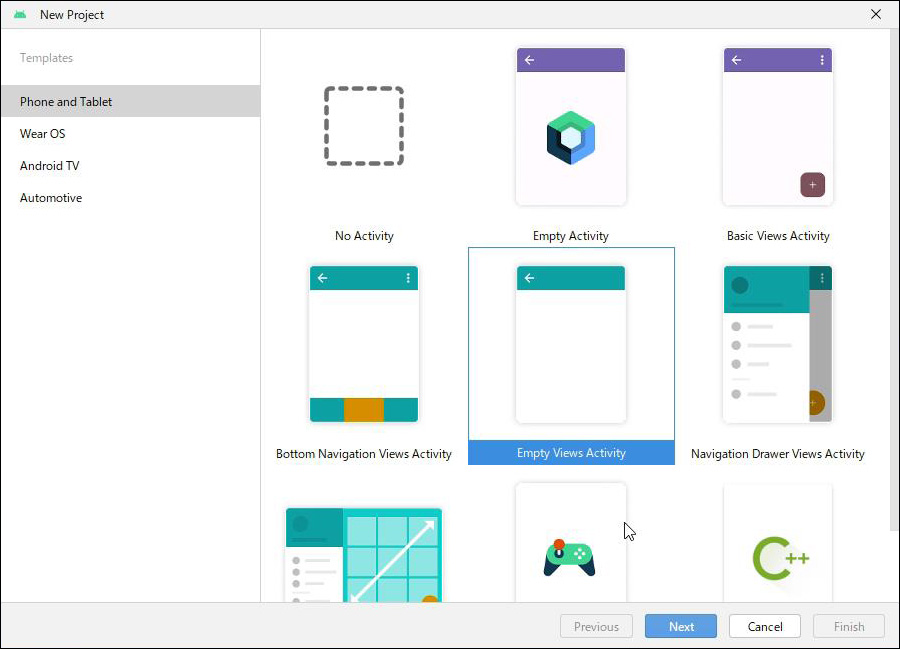
kotlinで、簡単な「足し算」ができる計算アプリの作り方を解説します。数字を入力して、ボタンを押すと、合計が表示される、というものです。
前回、前々回と解説してきたアプリはですね、TextViewとか、配列とかであらかじめ用意したワード・情報が表示される、というプログラミングを作ってきました。が、今回は、情報そのものをユーザに入力してもらおうと。で、ただ、表示させるだけじゃなくて、それらを計算して、表示させよう、というものです。
今回の主な学習のテーマはEditTextです。文字や数字を入力してもらうときには、EditTextを使います。ちなみに、この時の型は、String型でもなければint型でもなく「Editabl型」「編集型」という、もう1個別の型になります。詳しくは後で説明しますが、なんとなく頭に入れておいてください。
動画
コード
▼activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/et1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:ems="10"
android:hint="数字を入力(1)"
android:inputType="number"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/et2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:ems="10"
android:hint="数字を入力(2)"
android:inputType="number"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/et1" />
<Button
android:id="@+id/btnAdd"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="たす"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/et2" />
<TextView
android:id="@+id/tvAns"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:text="答え"
android:textSize="34sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/btnAdd" />
<Button
android:id="@+id/btnClear"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="24dp"
android:text="clear"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
▼MainActivity.kt
package com.example.addapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.TextView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
//1)idの取得(Viewを取得しますよ、idで)
val et1:EditText = findViewById(R.id.et1)
val et2:EditText = findViewById(R.id.et2)
val btnAdd:Button = findViewById(R.id.btnAdd)
val tvAns:TextView = findViewById(R.id.tvAns)
val btnClear:Button = findViewById(R.id.btnClear)
//2)クリック処理(足し算)
btnAdd.setOnClickListener {
//EditText(編集)型 ⇒ String(文字)型 ⇒ int(整数)型
val sum = et1.text.toString().toInt() + et2.text.toString().toInt()
tvAns.text ="合計は$sum"
}
//3)クリア処理
btnClear.setOnClickListener {
et1.text.clear()
et2.text.clear()
tvAns.text = "答え"
}
}
}