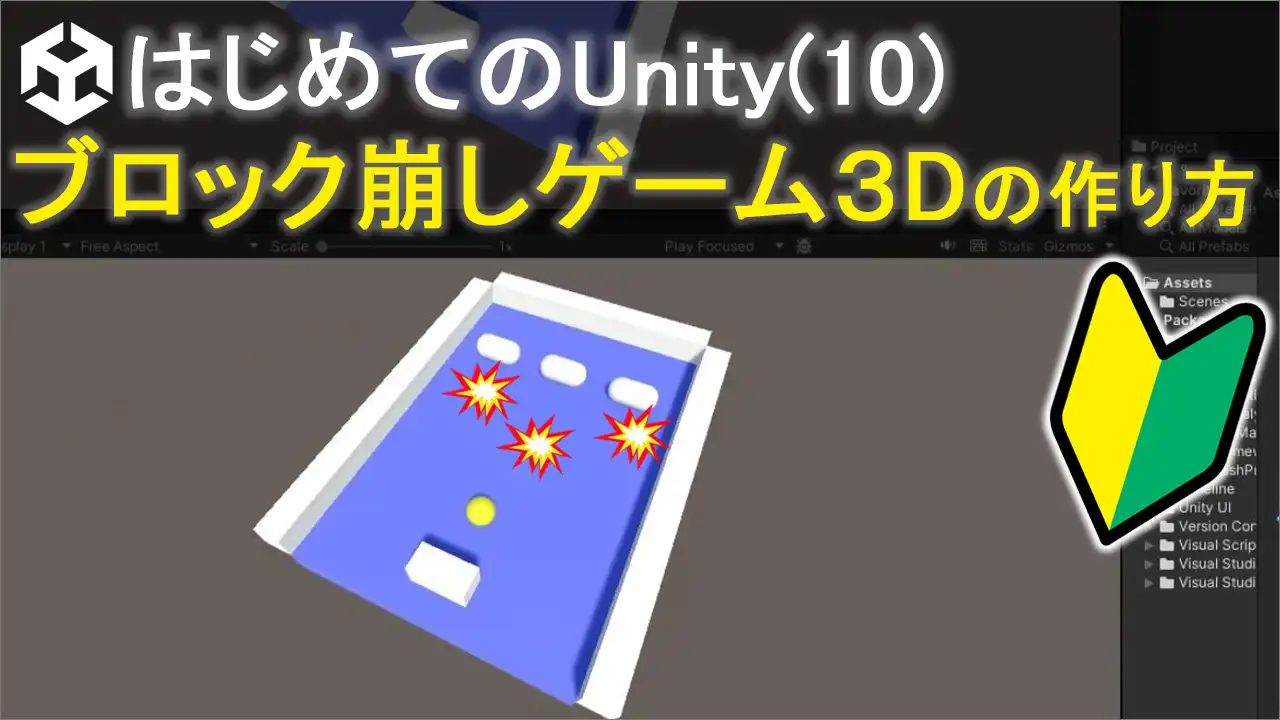
unityで、ブロック崩しゲーム3Dの作り方を解説したいと思います。
コード
▼BallScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallScript : MonoBehaviour
{
//1)Rigidbodyが扱える変数を用意
private Rigidbody rb;
// Start is called before the first frame update
void Start()
{
//2)Rigidbodyを取得して代入
rb = GetComponent<Rigidbody>();
//3)右奥方向に力を加える(x,z軸)
//[3-1]力を加える方向を決める
Vector3 myDirection = new Vector3(5, 0, 5);
//rb.AddForce(力を加える方向, 力の加えるモード);
rb.AddForce(myDirection, ForceMode.Impulse);// [3-2]インパルスモードで瞬間的に力を加える
}
// Update is called once per frame
void Update()
{
}
}
▼BarScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BarScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//1)左右の入力を取得(左が押されたのか、右が押されたのか)
float myX = Input.GetAxis("Horizontal");
//2)位置情報の変更
//transform.position += new Vector3(x, y, z);
transform.position += new Vector3(myX * 5, 0, 0) * Time.deltaTime;
}
}
▼BlockScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BlockScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
//1)当たったら
private void OnCollisionEnter(Collision collision)
{
Destroy(gameObject);//ここでのgameObjectはBlockのこと
}
}