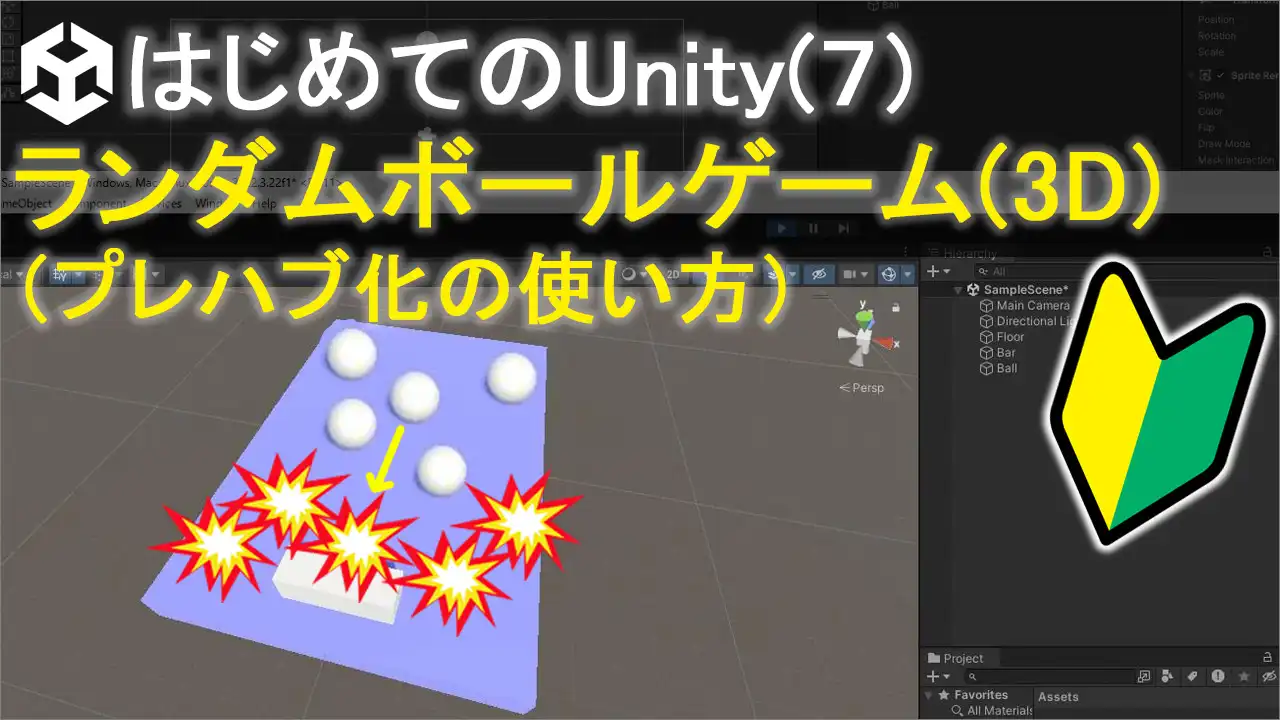
unityで、ランダムにボールが向かってきて バーに当たったらボールは消える、 当たらなければボールは下で落ちていくっていう ランダムボールゲームを3Dで作っていきたいと思います。
ちなみに同じようなゲームを過去に2Dでもやっていますので、 興味のある方はそちらもご覧ください。
今回はこの3D版です。 てことで、今回の主な学習のテーマは「プレハブ化」です。 前回までの動画で、ボールを1個だけ作って、それが当たったら消えるとか、 バー操作でよけるみたいな解説動画を作りました。 が、今回のように、ボールが何回も落ちてくる、みたいな同じ動作を繰り返す時には 同じボールを何個も何個も作るわけにはいかないので、 1つだけメインとなるテンプレートを作っておいて、 それをプログラムで毎回呼び出してあげることで 何回もリピートしてくれるので便利です。
【もくじ】
01:50 [1]オブジェクトの配置
11:10 [2]バーを左右移動
22:40 [3]ボールの移動
27:30 [4]当たったら消える
35:05 [5]ボールをプレハブ化
42:55 [6]リピート
コード
▼BarScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BarScript : MonoBehaviour
{
//3)スピードの数値を入れる変数を用意
public float spd;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//1)左右の入力を取得(左が押されたのか、右が押されたのか)
float myX = Input.GetAxis("Horizontal");
//2)移動の呪文(位置の移動)
//transform.position += new Vector3(x, y, z);
//transform.position += new Vector3(myX, 0f, 0f)* Time.deltaTime;
transform.position += new Vector3(myX * spd, 0f, 0f) * Time.deltaTime; //4)スピードをかける
}
}
▼BallScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//1)移動の呪文(位置情報の変更)
//transform.position += new Vector3(x, y, z);
transform.position += new Vector3(0f, 0f, -5f)*Time.deltaTime;
}
//2)当たったら
private void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.CompareTag("BarTag"))
{
Destroy(gameObject); //ここでのgameObjectはBallのこと
}
}
}
▼BallPrefabScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallPrefabScript : MonoBehaviour
{
//1)(インスペクターから)ボールを取得するための変数を用意
public GameObject balls;
// Start is called before the first frame update
void Start()
{
//2)ボールを1個生成してみる
//Instantiate(何を, 位置, 回転);
//Instantiate(balls, transform.position, transform.rotation);
//4)繰り返し処理
//InvokeRepeating("実行する関数名", 開始時間, 間隔);
InvokeRepeating("MakingBall", 0f, 2f);
}
// Update is called once per frame
void Update()
{
}
//3)ボール落下の関数
void MakingBall()
{
//Instantiate(何を, 位置, 回転);
Instantiate(balls,
new Vector3(Random.Range(-5f,5f),transform.position.y,transform.position.z),
transform.rotation);
}
}