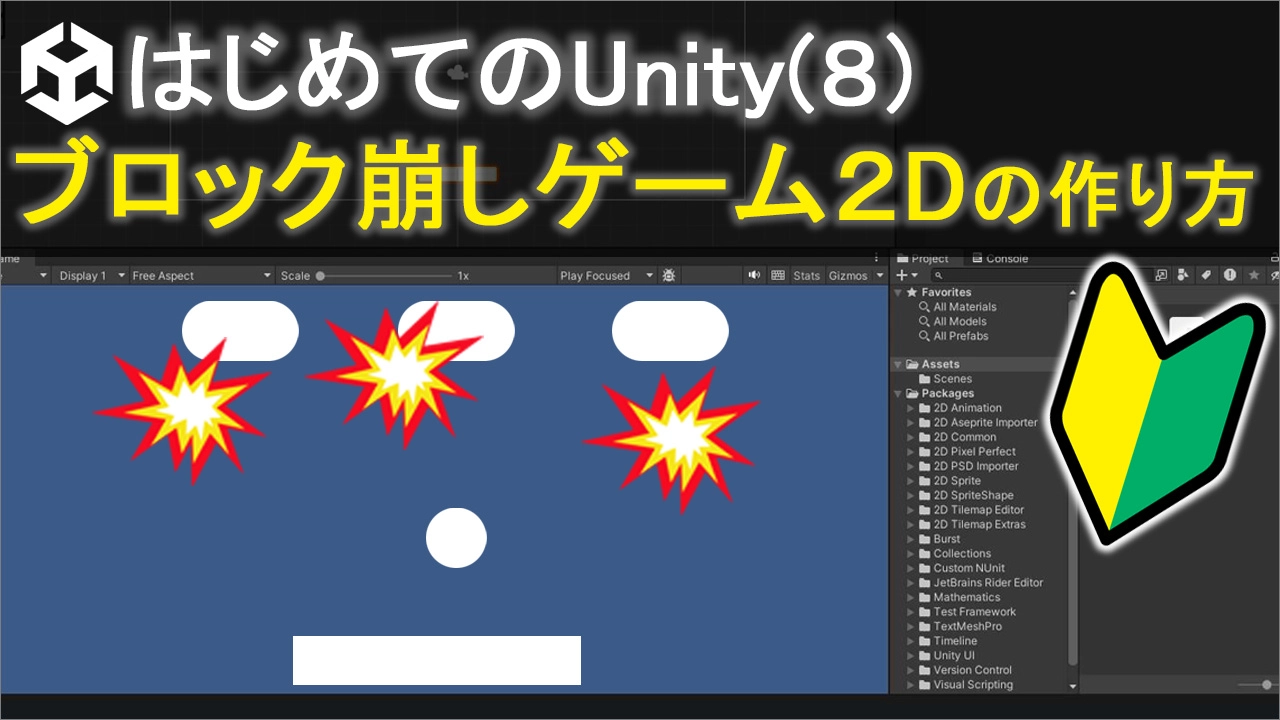
unityで、ブロック崩しゲーム2Dの作り方を解説したいと思います。 以前、いくつかの動画で、バー操作や、当たったら消える、みたいな 基本動作の解説をしてきました。 今回はそれらの知識をつかって、簡単なゲームを作っていきたいと思います。
今回の主な学習のテーマは
・当たり判定
・バウンド そして、
・物理シミュレーションのRigidbody
になります。 当たったら跳ね返るにはどうするか 当たったら消えるにはどうするか そして、ボールが特定の方向に動いてくれるにはどうするか みたいなのをゲームを作りながら解説していきたいと思います。
code
▼BallScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallScript : MonoBehaviour
{
//1)Rigidbodyが扱える変数を用意
private Rigidbody2D rb;
// Start is called before the first frame update
void Start()
{
//2)Rigidbodyを取得して代入
rb = GetComponent<Rigidbody2D>();
//3)右上方向に力を加える
//[3-1]力を加える方向
Vector2 myDirection = new Vector2(5, 5);
//rb.AddForce(力を加える方向, 力を加えるモード);
rb.AddForce(myDirection, ForceMode2D.Impulse);// [3-2]瞬間的に力を加える
}
// Update is called once per frame
void Update()
{
}
}
▼BarScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BarScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//1)左右の入力を取得(左が押されたのか、右が押されたのか)
float myX = Input.GetAxis("Horizontal");
//2)位置情報の変更
//transform.position += new Vector3(x, y, z);
transform.position += new Vector3(myX * 10, 0, 0) * Time.deltaTime;
}
}
▼BlockScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BlockScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
//1)当たったら
private void OnCollisionEnter2D(Collision2D collision)
{
Destroy(gameObject);//ここでのgameObjectはBlockのこと
}
}