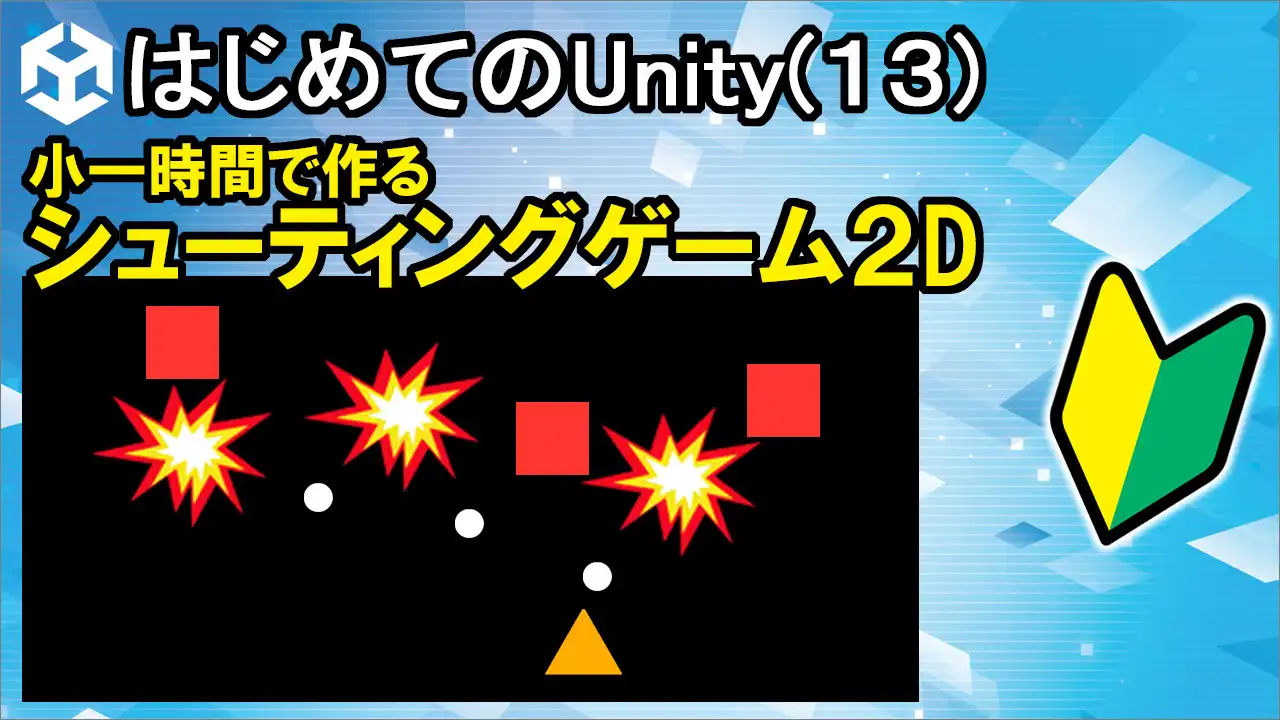
unityで、簡単なシューティングゲームを2Dを作ってみたいと思います。
-----------------------------------------------------------------
01:30 [1]オブジェクトの配置(プレイヤー、弾、敵)
16:30 [2]プレハブ化(弾、敵)
18:05 [3]プレイヤーの操作
23:55 [4]スペースキーで弾を生成
31:35 [5]弾を動かす
38:05 [6]敵の落下
43:25 [7]敵の出現
54:35 [8]当たったら消える
1:00:35 [おまけ]プレイヤーと当たったら
-----------------------------------------------------------------
オブジェクトの設定
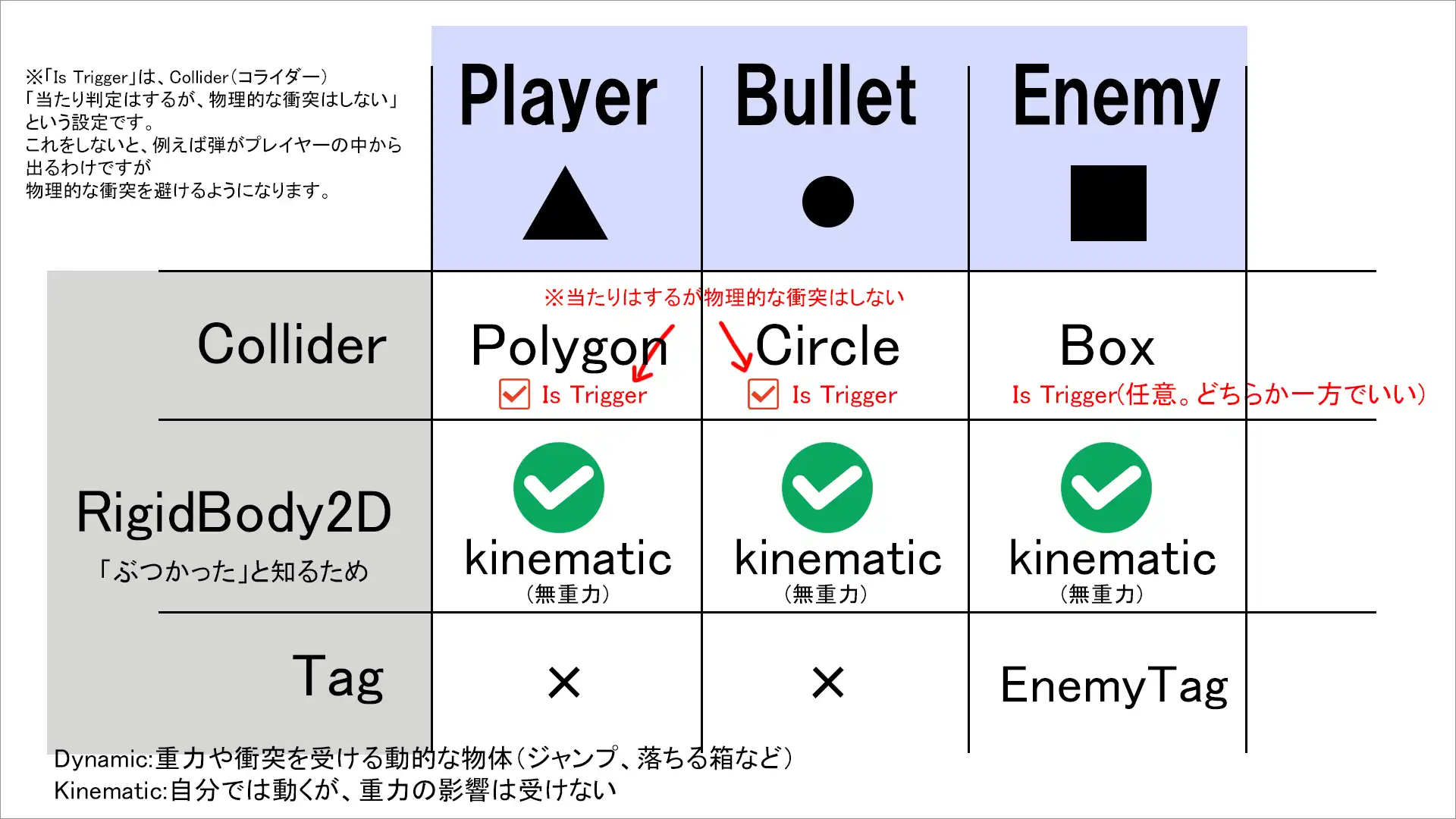
コード
▼PlayerScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerScript : MonoBehaviour
{
//3)弾のプレハブを定義
public GameObject bulletPrefab;
// Update is called once per frame
void Update()
{
// 1)入力を取得(←→キー、↑↓キー)
float moveX = Input.GetAxis("Horizontal"); // 左右
float moveY = Input.GetAxis("Vertical"); // 上下
//2)移動実行
//transform.position(座標) = 絶対移動(場所指定)←やり方によってはワープに近い
//transform.Translate(Vector3 移動量) は「現在の位置からの相対移動」
transform.Translate(new Vector3(moveX, moveY, 0f) *10f * Time.deltaTime);
//4)スペースキーで弾生成
if (Input.GetKeyDown(KeyCode.Space))
{
//Instantiate(何を, 位置, 回転);
//「回転を表す値(Quaternion)クオータニオン」の 初期状態(=回転していない状態)
Instantiate(bulletPrefab, transform.position + Vector3.up * 0.5f, Quaternion.identity);
}
}
//5)敵に当たったらプレイヤー破壊
private void OnTriggerEnter2D(Collider2D collision)
{
if(collision.tag == "EnemyTag")
{
Destroy(gameObject); //自分自身を消す
Time.timeScale = 0f; //ゲームを止める
Camera.main.backgroundColor = Color.red;// 背景色を赤に変更
}
}
}
▼BulletScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BulletScript : MonoBehaviour
{
// Update is called once per frame
void Update()
{
//1)弾が上に動いていく
transform.Translate(Vector3.up *10f * Time.deltaTime);
// 2)画面外で削除
if(transform.position.y > 7f)
{
Destroy(gameObject);
}
}
//3)当たったら(例:OnCollisionEnter2D)
private void OnTriggerEnter2D(Collider2D collision)
{
if(collision.tag == "EnemyTag")
{
Destroy(collision.gameObject); //当たった相手
Destroy(gameObject); //自分自身
}
}
}
▼EnemyScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyScript : MonoBehaviour
{
// Update is called once per frame
void Update()
{
//1)下に落下してくる
transform.Translate(Vector3.down * 2f *Time.deltaTime);
//2)下まで行ったら削除
if(transform.position.y < -6f)
{
Destroy(gameObject);
}
}
}
▼GameManager
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GameManager : MonoBehaviour
{
//1)敵のプレハブを定義
public GameObject enemyPrefab;
// Start is called before the first frame update
void Start()
{
//3)InvokeRepeating("実行する関数名", 開始時間, 間隔);
InvokeRepeating("CreateEnemy", 1f, 3f);
}
//2)敵出現の関数
void CreateEnemy()
{
float enemyX = Random.Range(-12f,12f);
Vector3 enemyPos = new Vector3(enemyX, 6f,0); //敵を生成する座標
//Instantiate(何を, 位置, 回転);
Instantiate(enemyPrefab, enemyPos, Quaternion.identity);
}
}
【補足】Quaternion.identity(初期角度)とtransform.rotation(オブジェクトの回転と同じ)、どう使い分けるか
今回、 Instantiate(何を, 位置, 回転);
のところで、角度の値を
「Quaternion.identity」(初期角度、回転なし。0度)
を使用しました。が、以前、ブロック崩しゲームの時には
「transform.rotation」(オブジェクトの回転と同じ)
を使用しました。
結論を言うと、どちらもエラーは起きません。よって、今回「transform.rotation」でも問題はないと思われます。
ただし意味が違ってくる
「回転無し」と「オブジェクトの回転と同じ」では意味がかわってきます。
どういう時に使い分けるか。少しまとめました
Quaternion.identity 初期角度、回転なし。0度 |
transform.rotation オブジェクトの回転と同じ |
●敵が毎回まっすぐ出てきてほしい(固定方向で出現) ●プレハブの回転角度に左右されたくない ●敵のスポーン処理を「どこからでも一定方向」で行いたい |
●スポーンする位置(発射台や砲台)が回転していて、その向きに合わせて敵や弾を出したい ●例えば、プレイヤーの向きを継承したいときや、斜めに出したいとき |