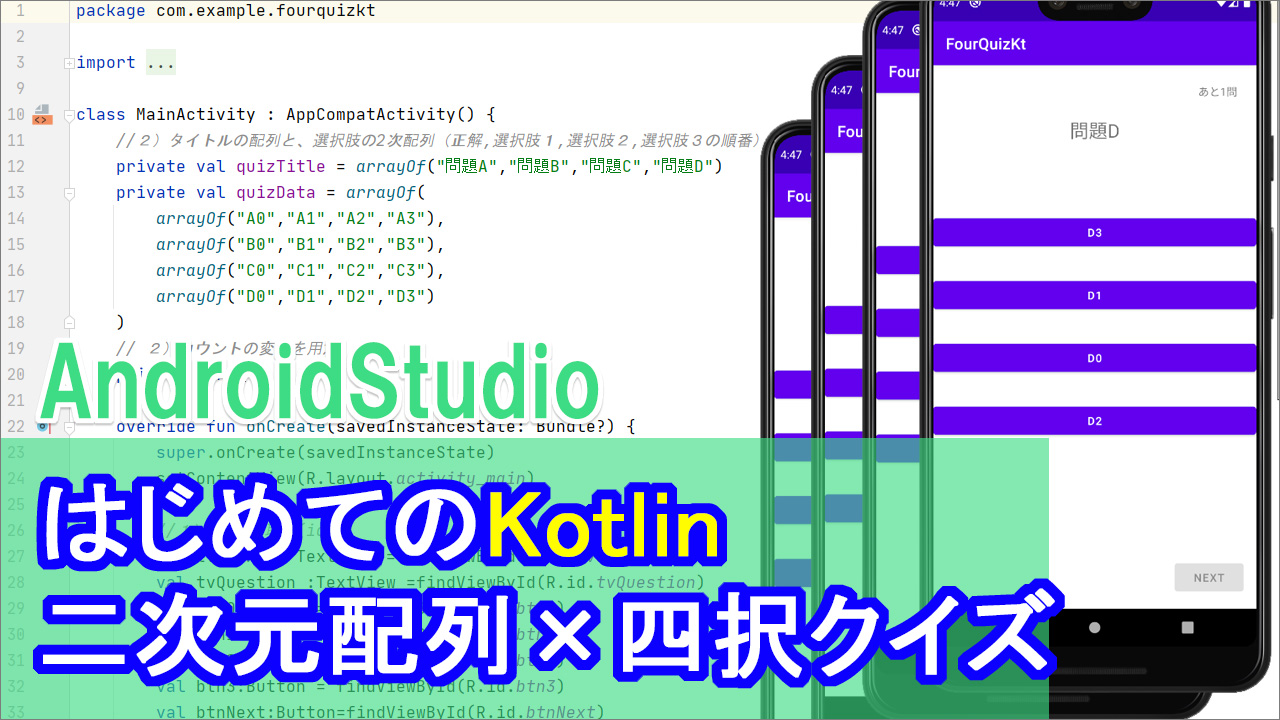
前回、1次元配列を使った四択クイズアプリをつくりました。
今回は、配列の中に配列を入れる多次元配列(2次元配列)を使って、
四択クイズアプリを作っていきましょう
動画
コード
▼activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/tvCount"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:layout_marginEnd="24dp"
android:layout_marginRight="24dp"
android:text="1問目"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/tvQuestion"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:text="Question"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/tvCount" />
<Button
android:id="@+id/btn0"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="90dp"
android:text="Button"
app:layout_constraintTop_toBottomOf="@+id/tvQuestion"
tools:layout_editor_absoluteX="177dp" />
<Button
android:id="@+id/btn1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:text="Button"
app:layout_constraintTop_toBottomOf="@+id/btn0"
tools:layout_editor_absoluteX="157dp" />
<Button
android:id="@+id/btn2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:text="Button"
app:layout_constraintTop_toBottomOf="@+id/btn1"
tools:layout_editor_absoluteX="132dp" />
<Button
android:id="@+id/btn3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:text="Button"
app:layout_constraintTop_toBottomOf="@+id/btn2"
tools:layout_editor_absoluteX="153dp" />
<Button
android:id="@+id/btnNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:layout_marginBottom="16dp"
android:text="NEXT"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
▼MainActivity.kt
package com.example.fourquizkt
import android.content.Intent
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.TextView
import androidx.appcompat.app.AlertDialog
class MainActivity : AppCompatActivity() {
//2)タイトルの配列と、選択肢の2次配列(正解,選択肢1,選択肢2,選択肢3の順番)
private val quizTitle = arrayOf("問題A","問題B","問題C","問題D")
private val quizData = arrayOf(
arrayOf("A0","A1","A2","A3"),
arrayOf("B0","B1","B2","B3"),
arrayOf("C0","C1","C2","C3"),
arrayOf("D0","D1","D2","D3")
)
// 2)カウントの変数を用意
private var i = 0
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
//1)viewを取得(idで)
val tvCount : TextView =findViewById(R.id.tvCount)
val tvQuestion :TextView =findViewById(R.id.tvQuestion)
val btn0:Button = findViewById(R.id.btn0)
val btn1:Button = findViewById(R.id.btn1)
val btn2:Button = findViewById(R.id.btn2)
val btn3:Button = findViewById(R.id.btn3)
val btnNext:Button=findViewById(R.id.btnNext)
//3)カウント数と、最初の問題を表示
tvCount.text = "あと4問"
tvQuestion.text =quizTitle[0]
//4)0~3までのリスト用意⇒シャッフル
val list = listOf(0,1,2,3)
val num = list.shuffled()
//3)ボタンにquizDataを表示+NEXTボタンは無効化
//5)シャッフルしたnumを表示
btn0.text =quizData[0][num[0]]
btn1.text =quizData[0][num[1]]
btn2.text =quizData[0][num[2]]
btn3.text =quizData[0][num[3]]
btnNext.isEnabled =false
//6)btn0を押した時の正誤判定
btn0.setOnClickListener {
if(btn0.text==quizData[i][0]){
//正解
correctAns()
}else{
//不正解
incorrectAns()
}
}
//10)btn1~3も同じようにする
btn1.setOnClickListener {
if(btn1.text==quizData[i][0]){
//正解
correctAns()
}else{
//不正解
incorrectAns()
}
}
btn2.setOnClickListener {
if(btn2.text==quizData[i][0]){
//正解
correctAns()
}else{
//不正解
incorrectAns()
}
}
btn3.setOnClickListener {
if(btn3.text==quizData[i][0]){
//正解
correctAns()
}else{
//不正解
incorrectAns()
}
}
//11)Nextボタンを押したらi問目
btnNext.setOnClickListener {
i++
//もう1回シャッフル
val numNext =list.shuffled()
//i問目のタイトルと問題を表示
tvCount.text ="あと" + (4-i) + "問"
tvQuestion.text = quizTitle[i]
btn0.text =quizData[i][numNext[0]]
btn1.text =quizData[i][numNext[1]]
btn2.text =quizData[i][numNext[2]]
btn3.text =quizData[i][numNext[3]]
//ボタンを有効化する
btn0.isEnabled =true
btn1.isEnabled =true
btn2.isEnabled =true
btn3.isEnabled =true
btnNext.isEnabled =false
}
}
//9)正解の関数
private fun correctAns(){
val btn0:Button = findViewById(R.id.btn0)
val btn1:Button = findViewById(R.id.btn1)
val btn2:Button = findViewById(R.id.btn2)
val btn3:Button = findViewById(R.id.btn3)
val btnNext:Button=findViewById(R.id.btnNext)
//12)全問正解で結果画面へ
if(i==3){
val intent = Intent(this,ResultActivity::class.java)
startActivity(intent)
finish()
}else{
//8)正解アラートダイアログ
AlertDialog.Builder(this)
.setTitle("正解!")
.setMessage(quizData[i][0])
.setPositiveButton("OK",null)
.show()
btn0.isEnabled =false
btn1.isEnabled =false
btn2.isEnabled =false
btn3.isEnabled =false
btnNext.isEnabled =true
}
}
//9)不正解の関数
private fun incorrectAns(){
val tvQuestion :TextView =findViewById(R.id.tvQuestion)
val btn0:Button = findViewById(R.id.btn0)
val btn1:Button = findViewById(R.id.btn1)
val btn2:Button = findViewById(R.id.btn2)
val btn3:Button = findViewById(R.id.btn3)
val btnNext:Button=findViewById(R.id.btnNext)
//7)不正解+ボタンの無効化
tvQuestion.text ="不正解!!Game Over"
btn0.isEnabled =false
btn1.isEnabled =false
btn2.isEnabled =false
btn3.isEnabled =false
btnNext.isEnabled =false
}
}
▼activity_result.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FFEB3B"
tools:context=".ResultActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="全問正解!Game Clear!"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
▼ResultActivity.kt
package com.example.fourquizkt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class ResultActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_result)
}
}
テキスト解説
準備中