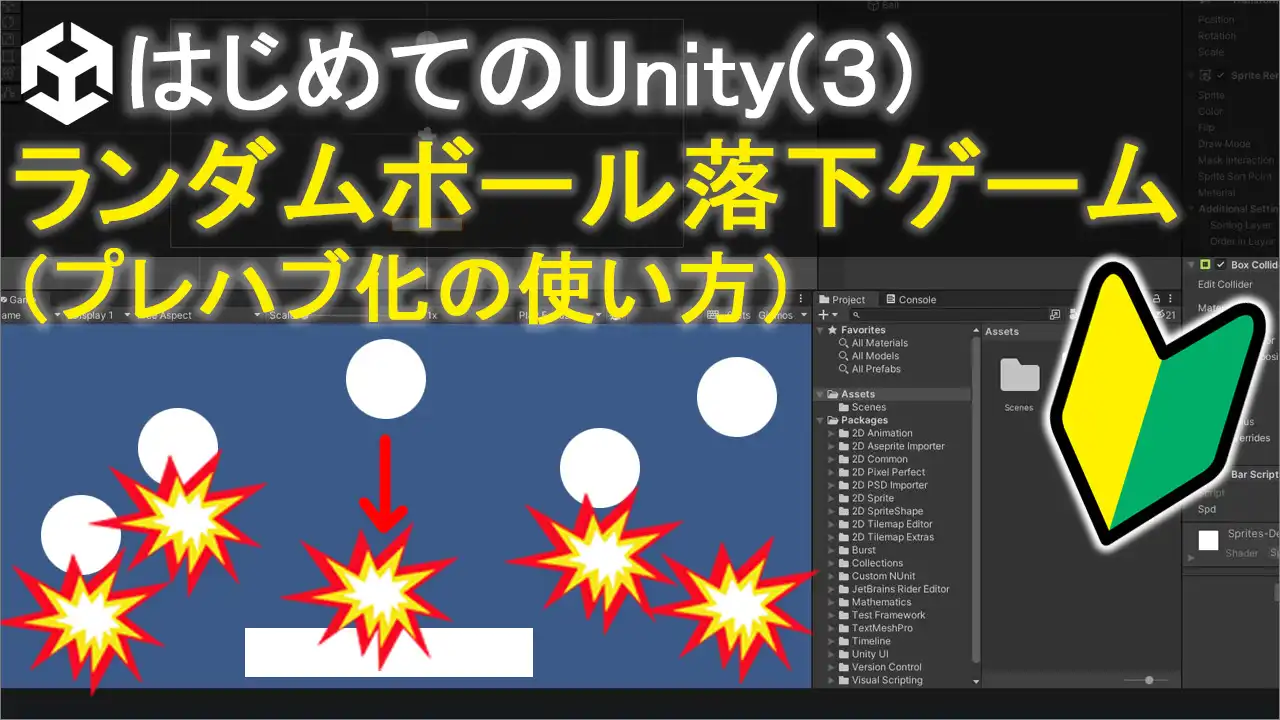
unityで、ランダムにボールが落ちてきて、 バーに当たったらボールは消える、 当たらなければボールは下で落ちていくっていう ランダムボール落下ゲームを作っていきたいと思います。
今回の主な学習のテーマは「プレハブ化」です。
今回のように、ボールが何回も落ちてくる、みたいな同じ動作を繰り返す時には 1つだけメインとなるテンプレートを作っておいて、 それをプログラムで毎回呼び出してあげることで 何回もリピートしてくれるので便利です。
コード
▼BallScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
//1)ボールが当たった時
private void OnCollisionEnter2D(Collision2D collision)
{
Destroy(gameObject);
}
}
▼BarScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BarScript : MonoBehaviour
{
//3)スピードの数値を入れる変数を用意
public float spd;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//1)左右の入力を取得(左が押されたのか、右が押されたのか)
float myX = Input.GetAxis("Horizontal");
//2)移動の呪文
//transform.position += new Vector3(x,y,z);
//transform.position += new Vector3(myX, 0, 0) * Time.deltaTime;
transform.position += new Vector3(myX * spd, 0, 0) * Time.deltaTime; //4)スピードをかける
}
}
▼BallPrefabScript
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallPrefabScript : MonoBehaviour
{
//1)(インスペクターから)ボールを取得するための変数を用意
public GameObject balls;
// Start is called before the first frame update
void Start()
{
//2)ボールを1個生成してみる
//Instantiate(何を, 位置, 回転);
//Instantiate(balls, transform.position, transform.rotation);
//4)繰り返し処理
//InvokeRepeating("実行する関数名", 開始時間, 間隔);
InvokeRepeating("FallingBall",0f, 2f);
}
// Update is called once per frame
void Update()
{
}
//3)ボール落下の関数
void FallingBall()
{
Instantiate(balls,
new Vector3(Random.Range(-11f,11f),transform.position.y,transform.position.z),
transform.rotation);
}
}