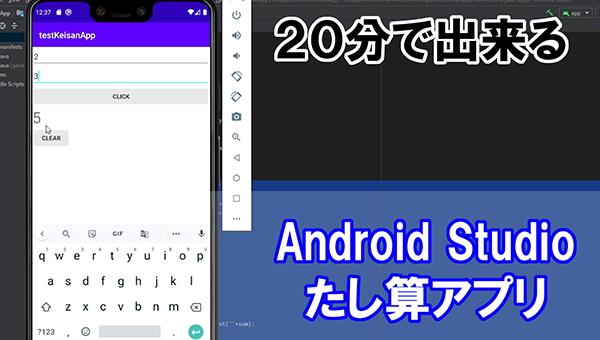
Android Studio(java)を使って、足し算ができる
簡単な計算アプリの作り方を解説実況します。
▼作業環境
OS:win10
AndroidStudio:4.0.2
言語:java
▼動画で実況解説(20分)
テキスト解説
▼xml(レイアウト)
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <EditText android:id="@+id/eT1" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="input a number(1)"/> <EditText android:id="@+id/eT2" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="input a number(2)"/> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:onClick="onButton" android:text="click" /> <TextView android:id="@+id/tvAns" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Answer" android:textSize="36sp" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="onClear" android:text="clear" /> </LinearLayout>
▼プログラミング(java)
import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.EditText; import android.widget.TextView; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void onButton(View view){ //Editの取得 EditText eT1 = (EditText)findViewById(R.id.eT1); EditText eT2 = (EditText)findViewById(R.id.eT2); //Edit⇒String String str1 = eT1.getText().toString(); String str2 = eT2.getText().toString(); //数値に変換 int num1= Integer.parseInt(str1); int num2= Integer.parseInt(str2); //足し算 int sum = num1 + num2; ((TextView)findViewById(R.id.tvAns)).setText("" + sum); } public void onClear(View view){ ((EditText)findViewById(R.id.eT1)).getText().clear(); ((EditText)findViewById(R.id.eT2)).getText().clear(); ((TextView)findViewById(R.id.tvAns)).setText("Answer"); } }
主なポイント
■point1:EditTextを文字型(String)に変換する
.getText().toString()
はよく使うので覚えておこう。
■point2:EditTextdで入力された情報を削除する「clear()」
最後のクリアボタンのところですね。情報を削除(クリア)するには
.getText().clear()
を使用します。
その他詳しくは動画をご覧ください